- 1 1. What is JSON? Understanding the Data Format from the Basics
- 2 2. JSON Basic Syntax and Features
- 3 3. Concrete Examples of JSON Usage
- 4 4. Handling and Processing JSON
- 5 5. JSON Applications and Latest Technologies
- 6 6. Comparison of JSON with Other Data Formats
- 7 7. The Future of JSON and Upcoming Trends
- 8 8. Conclusion and Additional Resources
- 9 FAQ: Frequently Asked Questions about JSON
- 9.1 1. What is JSON?
- 9.2 2. What are the typical use cases for JSON?
- 9.3 3. What data types are supported in JSON?
- 9.4 4. How is JSON different from XML?
- 9.5 5. What is the difference between JSON and YAML?
- 9.6 6. Which programming languages can use JSON?
- 9.7 7. Can comments be added to JSON?
- 9.8 8. How is error handling done in JSON?
- 9.9 9. Can JSON be converted to a binary format?
- 9.10 10. What are the latest trends and technologies in JSON?
- 9.11 Conclusion
1. What is JSON? Understanding the Data Format from the Basics
Definition and Basic Concepts of JSON
JSON is a text-based format primarily used to represent data as key-value pairs. This structure is very suitable for exchanging data between programs.
For example, user information can be represented in JSON as follows:
{
"name": "佐藤",
"age": 30,
"email": "sato@example.com"
}
In this example, the keys are “name”, “age”, and “email”, with corresponding values set for each. This makes it easy for humans to read and understand, and efficient for computers to process.
Features and Advantages of JSON
- Lightweight and Simple
- JSON is a compact format that doesn’t include unnecessary information, making it efficient for communication and data storage.
- Highly Readable
- Compared to formats like XML or binary, JSON is visually clearer and easier to debug and modify.
- Language Independent
- Since it’s supported by many programming languages, including JavaScript, it offers high compatibility and can be used for a wide range of applications.
- High Affinity with Object-Oriented Programming
- JSON is designed to be object-based, making it naturally compatible with object-oriented programming.
Background of JSON’s Popularity
Because JSON is originally based on JavaScript’s object syntax, it quickly became a standard format in Web application development.
Especially in data exchange with REST APIs, it has been widely adopted due to its simplicity and high compatibility. JSON requires less writing compared to XML and parsing is faster, making it suitable for mobile applications and cloud services.
Main Use Cases for JSON
- API Responses and Requests
- Widely used as a format for exchanging data between web services. For example, receiving data in JSON format is common with weather information APIs.
- Configuration Files
- JSON is used as a format for storing application settings. It is frequently used in configuration files (e.g., config.json).
- Data Storage and Databases
- In NoSQL databases (e.g., MongoDB), a JSON-based format is used as the data model.
- Data Analysis and Log Management
- Also utilized for data analysis and error log recording, where JSON’s structure facilitates parsing.
Summary
JSON is a lightweight and simple data exchange format, standardized and adopted in many systems including programming languages, databases, and APIs. Due to its readability and flexibility, it is a tool widely used by beginners and advanced users alike.
In the next section, we will delve deeper into the basic syntax of JSON and enhance understanding with practical code examples.
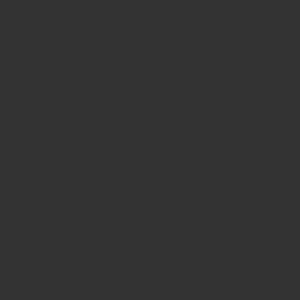
2. JSON Basic Syntax and Features
Basic Structure of JSON
JSON is composed of key-value pairs as its fundamental elements. Data is described using curly braces {}
as shown below.
Example: JSON representing user information
{
"name": "佐藤",
"age": 30,
"email": "sato@example.com",
"isMember": true
}
- Keys (e.g., “name”, “age”) must always be represented as strings.
- Values can be a string, number, boolean, null, array, or object.
Data Types and Examples
- String
- Must be enclosed in double quotes (“”).
- Example:
"title": "Introduction to JSON"
- Number
- Integers and floating-point numbers can be used.
- Example:
"price": 1999, "discount": 9.5
- Boolean
- Specify either true or false.
- Example:
"isAvailable": true
- null
- Indicates the absence of a value.
- Example:
"nickname": null
- Array
- Represents a list of data using square brackets
[]
. - Example:
"tags": ["JSON", "Data Format", "Programming"]
- Object
- Nested objects can be defined within an object.
- Example:
"address": { "city": "Tokyo", "zip": "100-0001" }
Points to Note When Writing JSON
- Always Use Double Quotes
- Keys and strings must be enclosed in double quotes (“). Single quotes will result in an error.
- No Trailing Commas
- Adding a comma after the last element in an array or object will cause an error.
- Incorrect Example:
{ "name": "佐藤", "age": 30, }
- Comments Cannot Be Included
- JSON does not support comments. If you need to add explanations within the documentation, a separate README file or similar should be prepared.
Summary
JSON is a format that allows efficient data management using key-value pairs, arrays, and objects. Its simple structure and flexibility make it widely used for data exchange and storage.
3. Concrete Examples of JSON Usage
Data Exchange via API
JSON is widely used as a data exchange format between web applications, mobile applications, and servers. Especially in REST APIs, it is standardly adopted as the data format for requests and responses.
Example: User information retrieval API
- Request
GET /users/1 HTTP/1.1
Host: example.com
Accept: application/json
- Response
HTTP/1.1 200 OK
Content-Type: application/json
{
"id": 1,
"name": "佐藤",
"email": "sato@example.com",
"status": "active"
}
Usage as Configuration Files
JSON is also used as a configuration file for applications.
Example: Configuration file (config.json)
{
"server": {
"host": "localhost",
"port": 3000
},
"database": {
"user": "admin",
"password": "password123",
"dbname": "exampledb"
},
"logging": {
"level": "info",
"enabled": true
}
}
Data Communication Between Frontend and Backend
JSON is frequently used in asynchronous communication using AJAX or Fetch API.
Example: Data retrieval using Fetch API (JavaScript)
fetch('https://api.example.com/users/1')
.then(response => response.json())
.then(data => {
console.log(data.name); // Displays "佐藤"
})
.catch(error => console.error('Error:', error));
Usage in Databases (NoSQL)
NoSQL databases store and manage data in JSON format.
Example: Data storage in MongoDB
{
"_id": ObjectId("507f1f77bcf86cd799439011"),
"name": "田中",
"age": 28,
"skills": ["JavaScript", "Python"]
}
Summary
JSON is an essential format in many scenarios, including API communication, configuration files, databases, and log management.
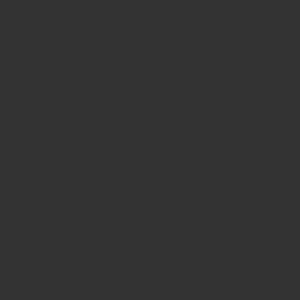
4. Handling and Processing JSON
Parsing and Generating JSON with JavaScript
Parsing JSON
const jsonString = '{"name": "田中", "age": 25, "isMember": true}';
const user = JSON.parse(jsonString);
console.log(user.name); // Output: 田中
Generating (Serializing) JSON
const user = {
name: "田中",
age: 25,
isMember: true
};
const jsonString = JSON.stringify(user, null, 2);
console.log(jsonString);
JSON Operations in Other Programming Languages
Python Example
import json
json_str = '{"name": "佐藤", "age": 28}'
data = json.loads(json_str)
print(data['name']) # Output: 佐藤
Error Handling
JavaScript Example
try {
const data = JSON.parse('{name: "佐藤"}'); // Error occurs
} catch (error) {
console.error('JSON Parsing Error:', error.message);
}
Summary
JSON allows for easy parsing and generation, enabling efficient data management.
5. JSON Applications and Latest Technologies
Data Validation with JSON Schema
JSON Schema is a specification for defining and validating the structure and format of JSON data.
Example: User information schema
{
"$schema": "http://json-schema.org/draft-07/schema#",
"title": "User Information",
"type": "object",
"properties": {
"name": {
"type": "string"
},
"age": {
"type": "integer",
"minimum": 0
},
"email": {
"type": "string",
"format": "email"
}
},
"required": ["name", "email"]
}
Structured Data with JSON-LD
JSON-LD provides data with semantic meaning for SEO optimization and search engines.
Example: Structured data for company information
{
"@context": "https://schema.org",
"@type": "Organization",
"name": "株式会社サンプル",
"url": "https://www.example.com",
"contactPoint": {
"@type": "ContactPoint",
"telephone": "+81-90-1234-5678",
"contactType": "customer service"
}
}
Latest Specifications and Extended Technologies
JSON5 Example
{
name: "田中", // Comments are allowed
age: 30,
skills: ["JavaScript", "Python",], // Trailing commas are OK
}
Summary
JSON can be widely applied for validation, SEO measures, and more.
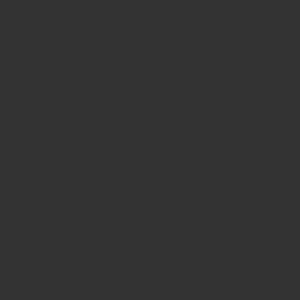
6. Comparison of JSON with Other Data Formats
Comparison of JSON and XML
Example: JSON
{
"user": {
"name": "佐藤",
"age": 30
}
}
Example: XML
<user>
<name>佐藤</name>
<age>30</age>
</user>
Comparison Table
Feature | JSON | XML |
---|---|---|
Syntax Simplicity | Simple and highly readable | Verbose with many tags |
Data Type Support | Supports numbers, booleans, etc. | Everything is treated as a string |
Parsing Speed | Fast | Comparatively slow |
Usage | Suitable for APIs and configuration files | Suitable for document management |
Comparison of JSON and YAML
Example: JSON
{
"name": "佐藤",
"age": 30
}
Example: YAML
name: 佐藤
age: 30
Comparison Table
Feature | JSON | YAML |
---|---|---|
Syntax Flexibility | Strict and simple | Closer to natural language and easy to read |
Comment Support | Not supported | Supported (using #) |
Usage | Suitable for programming and API communication | Suitable for configuration file management |
Summary
JSON has advantages in simplicity and speed, making it ideal for data management and API communication.
7. The Future of JSON and Upcoming Trends
JSON Standardization and Specification Evolution
JSON has been standardized in RFC 8259 and uses UTF-8 as the basic encoding.
Introduction of JSON5
An extended specification that adds flexible writing and comment support.
JSON5 Example
{
name: "田中",
age: 25, // Comments are allowed
}
JSON-LD and the Semantic Web
Supports SEO enhancement and data semantic annotation. Provides structured data for search engines.
Future Challenges
- Speed Improvement: Transition to binary formats like BSON.
- Enhanced Security: Development of escape processing and validation tools.
Summary
JSON continues to evolve by combining with the latest technologies, playing a central role in data management.
8. Conclusion and Additional Resources
Summary of Article Key Points
- JSON is widely adopted as a simple and efficient data exchange format.
- Its application range is wide, including API communication, configuration files, and SEO measures.
- Collaboration with the latest technologies and security measures are also evolving.
Introduction of Additional Resources
- JSON Official Website – JSON specification and basic rules.
- JSONLint – JSON syntax check tool.
- MDN Web Docs – Explanation of JSON in JavaScript.
Future Learning and Practical Advice
- Utilize JSON in small-scale projects.
- Learn advanced techniques such as JSON Schema and JSON-LD.
- Catch up on the latest trends in communities and forums.
Conclusion
JSON is a format that realizes efficient data management and expands its possibilities when combined with the latest technologies.
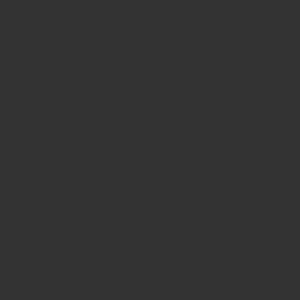
FAQ: Frequently Asked Questions about JSON
1. What is JSON?
A: JSON (JavaScript Object Notation) is a text-based data exchange format designed for simple and efficient data representation.
- It consists of key-value pairs, allowing for clear representation of data structure.
- It is lightweight and supported by many programming languages, primarily used in API communication and configuration files.
2. What are the typical use cases for JSON?
A: JSON is used in the following applications:
- API Communication: Used for sending and receiving data between clients and servers.
- Configuration Files: Stores configuration information for applications and tools.
- Databases: Used for data management in NoSQL databases (e.g., MongoDB).
- Log Management: Records error logs and debugging information.
- Structured Data: Used in JSON-LD format for SEO enhancement.
3. What data types are supported in JSON?
A: JSON supports the following six data types:
- String:
"name": "佐藤"
- Number:
"age": 30
- Boolean:
"isMember": true
- null:
"nickname": null
- Array:
"skills": ["JavaScript", "Python"]
- Object:
"address": {"city": "東京", "zip": "100-0001"}
4. How is JSON different from XML?
A: The differences between JSON and XML are as follows:
Feature | JSON | XML |
---|---|---|
Syntax Simplicity | Simple and highly readable | Verbose with many tags |
Data Type Support | Native support for numbers, arrays, etc. | Everything is treated as a string |
Parsing Speed | Fast | Comparatively slow |
Usage | Suitable for data transfer and configuration files | Suitable for document and structured data management |
5. What is the difference between JSON and YAML?
A: YAML is a data format that is easier for humans to read and write, and differs from JSON in the following ways:
Feature | JSON | YAML |
---|---|---|
Syntax Format | Strict and simple | Flexible and closer to natural language |
Comment Support | Comments not allowed | Comments can be included (using #) |
Usage | Suitable for program processing and data communication | Suitable for configuration file and configuration management |
6. Which programming languages can use JSON?
A: JSON is supported by many programming languages, including the following:
- JavaScript: Uses standard functions
JSON.parse()
andJSON.stringify()
. - Python: Uses the
json
module for parsing and generation. - PHP: Uses
json_decode()
andjson_encode()
functions. - Java: Uses libraries like
Jackson
orGson
.
7. Can comments be added to JSON?
A: No, comments are not supported in the standard JSON specification. However, when used as a configuration file, the following alternatives can be used:
- Add a comment-specific key:
{
"_comment": "This setting is for debugging",
"debug": true
}
- Use JSON5: Comments are supported in JSON5.
8. How is error handling done in JSON?
A: Examples of handling errors that occur during JSON processing are shown below.
JavaScript Example:
try {
const data = JSON.parse('{name: "佐藤"}'); // Error occurs
} catch (error) {
console.error('JSON Parsing Error:', error.message);
}
9. Can JSON be converted to a binary format?
A: Yes, it can. BSON (Binary JSON) is a specification that optimizes JSON into a binary format.
- It is mainly used in NoSQL databases such as MongoDB.
- Its characteristic is fast and efficient data processing.
10. What are the latest trends and technologies in JSON?
A: The latest technologies for JSON include the following:
- JSON-LD: Describes structured data and is used for SEO optimization.
- JSON5: An extended specification that adds flexible writing and comment support.
- GraphQL: An API design that flexibly retrieves data in JSON format.
- Serverless Architecture: Used for event-driven data processing in AWS Lambda, Google Cloud Functions, etc.
Conclusion
This FAQ covers everything from basic questions about JSON to advanced technologies.
While JSON is simple and easy to handle, situations may require dealing with syntax errors or extended specifications. Use this article and FAQ as a reference to master the basics and applications of JSON and utilize it in your actual projects.